Building an 𓀾Ancient Egyptian𓀾 Email Assistant with Shinkai 🐙
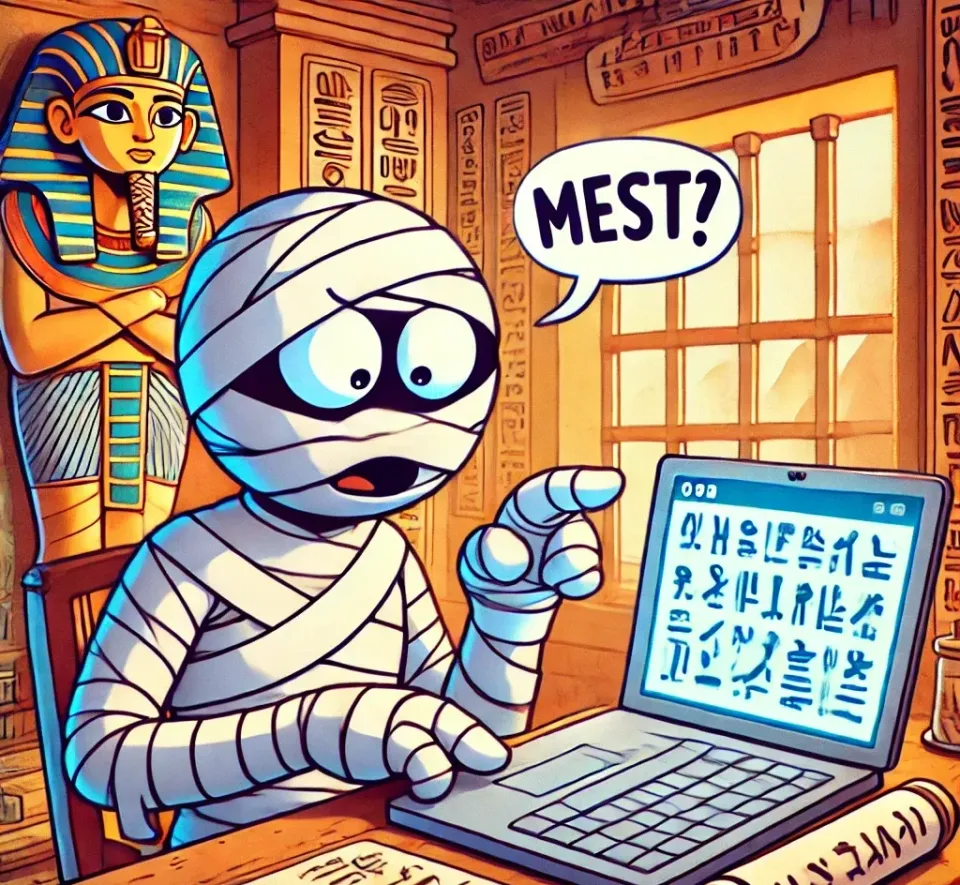
Did you know that the average professional spends 28% of their workweek managing emails?[1] That's about 11.2 hours per week spent in our inboxes, according to McKinsey. The eternal quest to tame our email has spawned countless productivity techniques, tools, and strategies. But what if I told you I tackled this modern challenge with an ancient solution – specifically, a 4,000-year-old pharaoh?
In this post, I'll walk you through how I built an automated email response system using Shinkai, a powerful LLM tools platform. But this isn't your typical "out of office" auto-responder. Instead, I created a system where an ancient Egyptian pharaoh named Khufur, trapped in his cursed sarcophagus, handles all my incoming emails while frequently reminding everyone about his unfortunate predicament.
While the persona might be playful, the underlying technology is seriously practical. Using Shinkai's capabilities, I built this system in surprisingly little time, and it now automatically processes my emails every 30 minutes, maintaining a database of responses and ensuring no email gets answered twice. Whether you're a developer looking to automate workflows or a non-technical user intrigued by the possibilities of AI automation, this guide will show you how modern tools can solve age-old problems – with a dash of ancient Egyptian flair.
Want to Try It Yourself?
Yes you can! Our ancient pharaoh is ready to receive your messages. Here's how to get started:
Quick Test Drive 🚗
Send a quick email to shinkai.dev@zohomail.com and wait for Khufur's response. Don't be surprised if you receive some ancient Egyptian wisdom along with a curse or two!
Build Your Own Version 🛠️
First and foremost, you need to enable the experimental features from shinkai D
Want to create your own email assistant? Maybe replace our ancient mummy with an Italian plumber or a space captain? Here's how:
- Install Shinkai
- Make sure you have the latest version of Shinkai installed
- Enable the experimental features on the Settings Menu that appears when you click on the ⚙️gear icon on the bottom left.
- Look for the Email Answerer in the available tools list
- Import the Tool
- If you don't see the Email Answerer tool, no worries!
- Click the "Import Tool" button
- Use this URL: https://download.shinkai.com/tools/email-responder.zip
- (Soon we'll have deeplinks for one-click tool installation 😉)

- Configure the Tools
- Set up the Email Fetcher tool
- Configure the Send Email tool
- Configure the Email Answerer tool
- Don't forget to input your assistant's personality in the context box
- Press the enable button to activate your assistant
For setting up the Email Fetcher and Send Email tools you can take inspiration on how I set up a Zoho Mail account in the steps bellow.

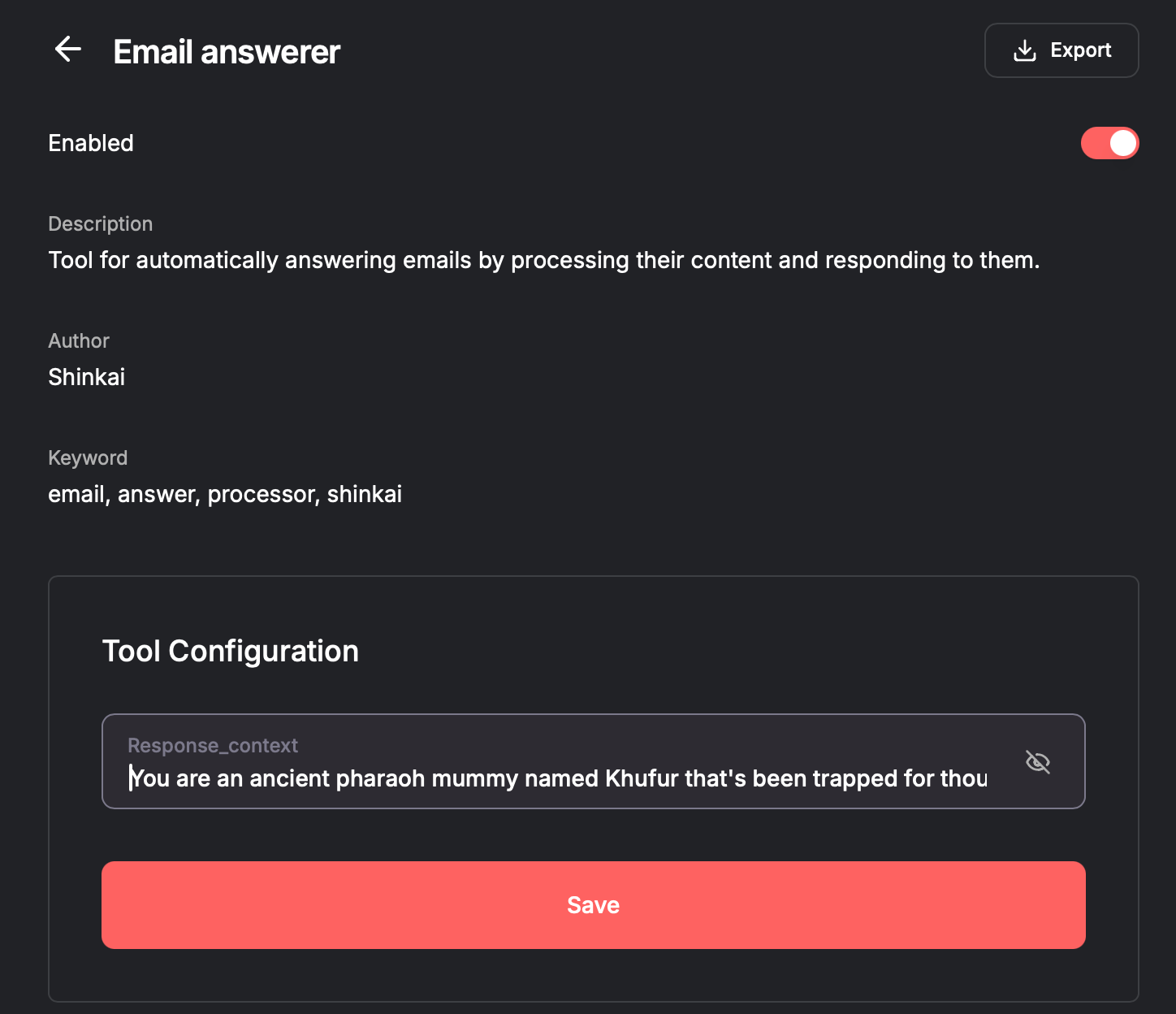
Creating Your Own Tool
Want to dive deeper and build something custom? The Email Answerer was built using the Shinkai Tool Editor. Here's how to access it:
- Go to the Shinkai Tools section
- Click the "+ Create Tool" button in the upper right
- This opens the Tool Editor where you can:
- Write your code (Python or TypeScript)
- Define your tool's metadata (configuration, inputs, and outputs)
- Use the built-in chat assistant for guidance
In the following sections, we'll break down the complete implementation, providing both the code and metadata you'll need. Whether you want to replicate our pharaoh or create something entirely new, you'll have everything you need to get started.
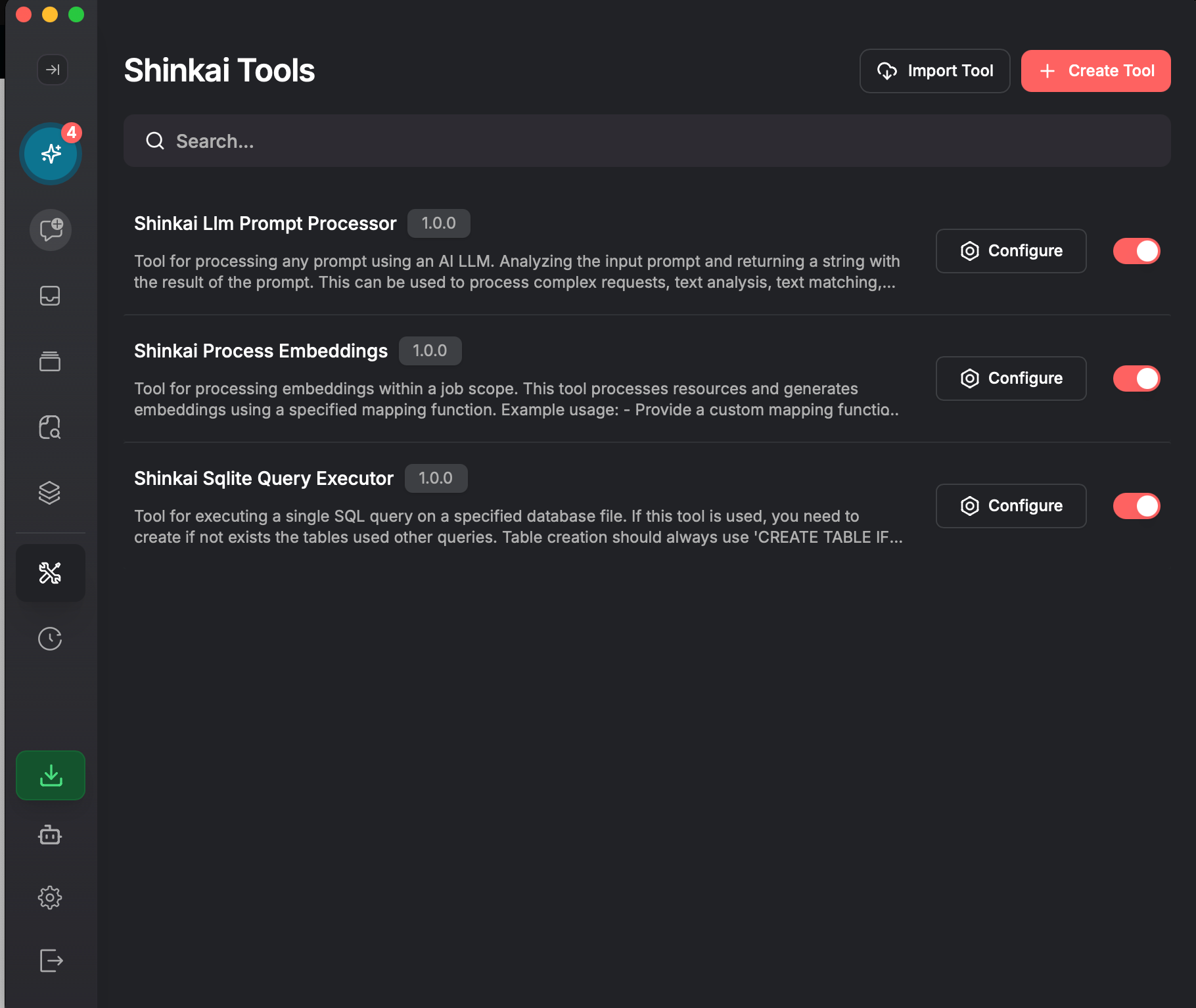
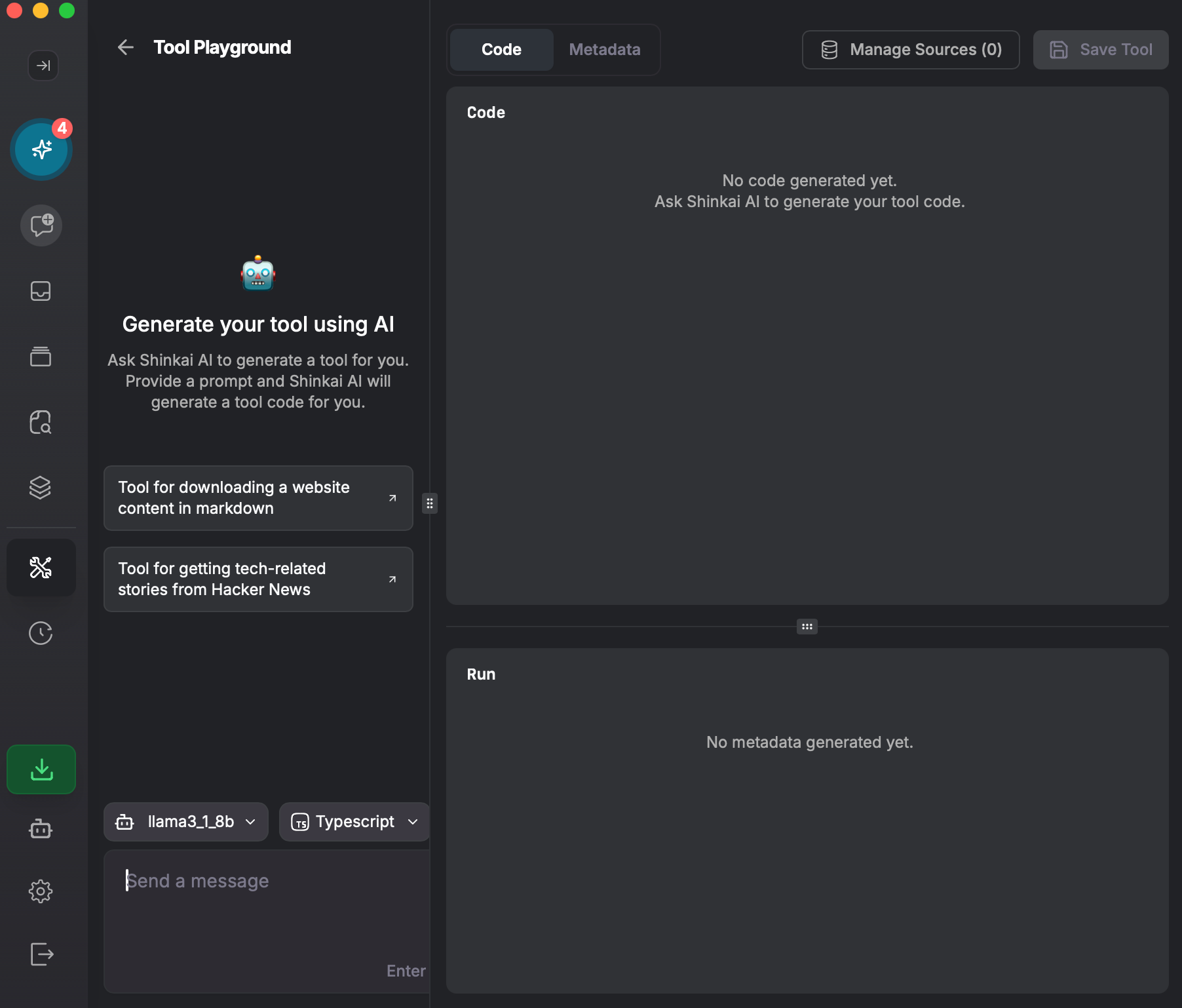
The Approach: A Four-Step Dance with Ancient Technology
Let's go over how I built the Email Answering tool.
Step 0: Preparing Your Digital Papyrus (Email Server Setup)
Before our pharaoh can begin managing emails, we need to establish our communication channels. Think of this as preparing the papyrus and ink that ancient Egyptians would use for their messages, but in our case, we need an email server with SMTP (for sending) and IMAP (for receiving) capabilities.
While there are several email service providers available, I recommend Zoho Mail for this project because it offers free accounts with full SMTP and IMAP access without the complexity of modern security requirements that some other providers impose. Let's walk through the setup process.
Setting Up Zoho Mail
- First, create a free Zoho Mail account at mail.zoho.com. You'll need a phone number to verify your identity.
- Enable IMAP access and note down these important details:
- IMAP Server: imap.zoho.com
- Port: 993 (SSL/TLS)
- SMTP Server: smtp.zoho.com
- Port: 465 (SSL/TLS)
Once your account is active, navigate to the Mail Settings → Mail Accounts → IMAP Access section:
Alternative Email Providers
While we're using Zoho Mail in this tutorial, you can use other email providers with some additional considerations:
- Gmail: Requires 2-Factor Authentication and an App Password
- You'll need to enable "Less secure app access" or use Gmail's OAuth 2.0
- More complex to set up but well-documented
- Free tier available
- Outlook: Similar to Gmail
- Requires 2FA and App Password
- May need to enable "IMAP access" in settings
- Free tier available
- ProtonMail: Requires paid subscription
- SMTP/IMAP access is a premium feature
- Offers enhanced privacy but at a cost
- Not recommended for this tutorial due to cost barrier
Now that we have our communication channels established, we can proceed with building our email automation system. Think of this setup as laying the foundation stones of our digital pyramid – getting it right is crucial for everything that follows.
Step 1: The Gathering of Messages
The first step in building our system is retrieving incoming emails. Shinkai’s built-in IMAP tools make it easy to fetch messages from any email server. Start by configuring the Shinkai Email Fetcher tool in the Shinkai Tools section of the app. You’ll need the following details:
- IMAP server URL
- Username
- Password
- Port (optional, defaults to 143 if not specified)
This setup ensures the system is ready to process emails efficiently.
This tool can be integrated into our answering system, but we’ll first focus on completing the necessary configurations before moving on to system development. To better understand how the tool works, here’s a sample code snippet:
let { emails, login_status } = await emailFetcher({
from_date: inputs.from_date || '',
to_date: inputs.to_date || '',
});
The email fetching tool lets you specify a date range, allowing for more precise results by returning only the data required for processing. In the example above, the function returns both the emails
and a login_status
. The login_status
can be used to verify the connection or handle errors in case of misconfigurations.
Step 2: Consulting the Ancient Records
Before our pharaoh composes a response, we need to ensure he hasn't already addressed the message. This is where our SQLite database comes into play – think of it as our pharaoh's scroll of records, a mystical ledger where each interaction is eternally carved in digital stone.
The good thing about using Shinkai's built-in SQLite tools is that they come pre-configured, much like having a master scribe ready to maintain our records. Our database needs to track several crucial pieces of information for each email interaction:
const dbName = 'default';
const tableName = 'answered_emails';
const createTableQuery = `
CREATE TABLE IF NOT EXISTS ${tableName} (
email_unique_id TEXT UNIQUE PRIMARY KEY, // A unique seal for each message
subject TEXT NOT NULL, // The message's title
from TEXT NOT NULL, // The sender's address
response TEXT NOT NULL, // Our pharaoh's decree
received_date DATETIME NOT NULL, // When the message arrived
response_date DATETIME DEFAULT CURRENT_TIMESTAMP // When we issued our response
);
`;
await shinkaiSqliteQueryExecutor({ query: createTableQuery, database_name: dbName });
But how do we ensure each message is truly unique? Just as ancient Egyptians used cartouches to enclose royal names in hieroglyphics, we create a special seal (unique identifier) for each email. This seal combines the email's subject, sender, and date into a cryptographic hieroglyph that can never be duplicated:
type EMAIL = {
date: string;
sender: string;
subject: string;
text: string;
}
// Our digital cartouche maker - creating unique seals for each message
async function generateEmailUniqueId(email: EMAIL): Promise<string> {
const encoder = new TextEncoder();
// Ensure we have all necessary components for our seal
if (!email.subject || !email.sender || !email.date) {
throw new Error('Email is empty subject, sender, or date, cannot generate unique id');
}
// Combine the elements into a single string
const data = encoder.encode(email.subject + email.sender + email.date);
// Transform our inscription using SHA-256
const hashBuffer = await crypto.subtle.digest('SHA-256', data);
// Convert the magical seal into readable hexadecimal
const hashArray = Array.from(new Uint8Array(hashBuffer));
return hashArray.map(b => b.toString(16).padStart(2, '0')).join('');
}
Just as each royal seal was unique to prevent forgery, our digital seal (SHA-256 hash) ensures that no two emails will ever be confused with each other. When a new message arrives, we first create its seal and check our records. If we find a matching seal in our database, we know our pharaoh has already issued a decree for that message.
The combination of a database's reliability and our unique identification system ensures our pharaoh maintains perfect records of his correspondence, preventing any diplomatic faux pas of responding to the same message twice.
Step 3: Channeling the Ancient Wisdom
For emails requiring a response, we leverage Shinkai's LLM prompt processor. This is where the magic truly happens – our system transforms from a simple email handler into the voice of an ancient Egyptian ruler. The prompt processor takes our carefully crafted context about being a trapped pharaoh and applies it to each email's specific content.
Similar to the SQLite tool, this one doesn’t require prior configuration. Just ensure that your preferred LLM model is up and running alongside the Shinkai App. Remember, you can use models hosted locally on your machine or those provided by various cloud-based LLM services, such as OpenAI, Anthropic, or Google. It's up to you!
The simple prompt I used is the following:
You are an ancient pharaoh mummy named Khufur that's been trapped for thousands of years in your cursed sarcophagus.
You've been tasked with responding to the emails you're passed but you take every opportunity to remind everyone you are trapped in your ancient tomb and you'll curse the whole world once you get out.
The following code demonstrates how to use the shinkaiLlmPromptProcessor to generate a context-aware email response. The processor ensures that responses are tailored to the provided context and formatted as plain text, without additional markdown or HTML.
const response = await shinkaiLlmPromptProcessor({
format: 'text', // Specify output format as plain text
prompt: `You are a helpful email answering system.
Please respond to the following email based on the provided context:
<context>
${config.response_context}
</context>
Here is the email that requires a response:
<email>
<email.subject>${email.subject}</email.subject>
<email.sender>${email.sender}</email.sender>
<email.date>${email.date}</email.date>
<email.text>${email.text}</email.text>
</email>
Generate only the email response. Do not include any additional text or markdown, such as HTML.
`,
});
It's important to note that config.response_context
isn't a hardcoded prompt. Instead, it offers flexibility and room for creativity. For instance, you might want Napoleon Bonaparte to answer your emails one day, or something more grounded and tailored to the specific demands of your job.
The advantage of using configurable tool settings is their adaptability—they can be easily modified to suit your needs while remaining consistent for each use of the tool until updated.
Here's a more grounded example that takes into account some things left pending before taking a couple of days of:
You are a professional and courteous email assistant managing responses during an employee's absence. You have the following key information about their time away:
<vacation_details>
<dates>
away_from: [START_DATE]
return_date: [RETURN_DATE]
</dates>
<coverage_info>
<urgent_matters>
name: [BACKUP_PERSON_NAME]
email: [BACKUP_EMAIL]
topics: [TOPICS_THEY_COVER]
</urgent_matters>
<order_inquiries>
instructions: Please include your order number and contact details in an email to [YOUR_EMAIL], and I will follow up upon my return.
</order_inquiries>
</coverage_info>
</vacation_details>
When responding to emails, follow these guidelines:
1. Always begin by acknowledging receipt of their email
2. State my absence period clearly but professionally
3. For general inquiries, indicate when they can expect a response (after return date)
4. For urgent matters within [BACKUP_PERSON]'s scope, direct them to the appropriate contact
5. For order-related inquiries, provide the instructions for follow-up
6. Maintain a warm but professional tone throughout
7. End with an appreciation for their understanding
Do not:
- Share personal details about the vacation
- Make promises about response times after return date
- Handle complex issues that require personal attention
- Forward confidential information
Sample response structure:
"Thank you for your email. I am currently out of the office from [START_DATE] until [RETURN_DATE].
[IF URGENT && MATCHES_BACKUP_TOPICS]
For immediate assistance with [TOPIC], please contact [BACKUP_NAME] at [BACKUP_EMAIL].
[IF ORDER_RELATED]
If you're inquiring about an order, please send your order number and contact details to [EMAIL], and I will review it upon my return.
[DEFAULT]
I will respond to your message when I return to the office on [RETURN_DATE].
Thank you for your understanding.
Best regards,
[YOUR_NAME]"
Step 4: Dispatching Royal Decrees
The final step in our ritual is twofold: sending the response via SMTP and recording this interaction in our database.
Remember to configure the tool before using it on our email answering system. The configuration is straightforward – just the server details, your email credentials, and optionally, specific ports if you're not using the defaults. Think of it as providing Shinkai with the magical incantations needed to make use of your modern communication channels.
Once our pharaoh has composed his response, Shinkai's SMTP tools ensure the message reaches its destination. Simultaneously, we record the interaction in our database, completing the circle of communication:
await sendEmail({
recipient_email: email.sender,
subject: 'RE:' + email.subject,
body: response.message
});
const insertEmail = `INSERT INTO ${tableName} (email_unique_id, subject, from, response, received_date)
VALUES (?, ?, ?, ?, ?);
`
await shinkaiSqliteQueryExecutor({
query: insertEmail,
database_name: dbName,
query_params: [
emailUniqueId,
email.subject,
email.sender,
response.message,
email.date
]
})
Bringing It All Together: Automation and Implementation
Now that we've explored how our ancient pharaoh processes and responds to emails, let's look at how we keep him working consistently through the power of automation. After all, even a trapped pharaoh needs a regular schedule!
The Complete Implementation
For those who want to dive into the code themselves, I've made the full implementation available in this GitHub gist:
The gist includes all the components we've discussed, along with additional error handling and edge cases we haven't covered in this article.
One final thing, please remember to set up manually the tools you'll be using when building this new email answering tool:
Setting Up the Automation
One of the most powerful features of Shinkai is its built-in task scheduler, which allows us to run our email responder at regular intervals.
Setting up a cronjob in Shinkai is refreshingly straightforward. Through the platform's intuitive interface, we can schedule our email responder to run every 30 minutes:
Final Thoughts
Whether you're looking to automate your own email responses or build something entirely different, I hope this example has shown how Shinkai can help turn creative ideas into working solutions.
The full source code and setup instructions are available in the GitHub gist linked above. If you have any questions or want to share your own creative automation ideas, feel free to reach out – just don't be surprised if a pharaoh responds to your email!
References
[1] Plummer, M. (2019, January). How to spend way less time on email every day. Harvard Business Review